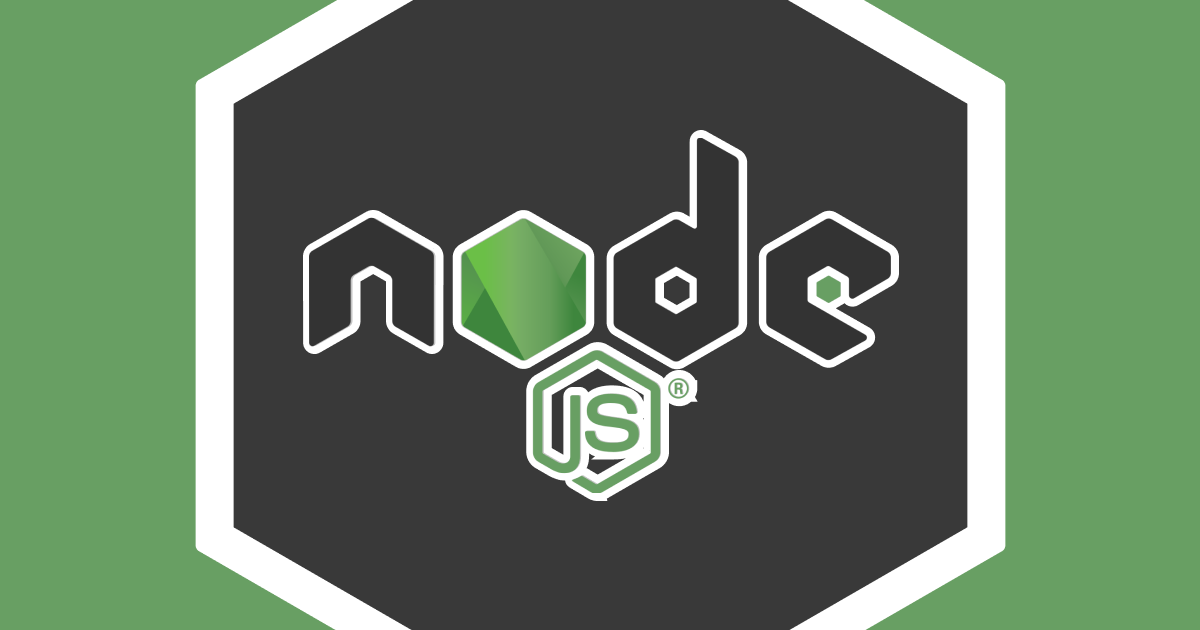
Initializing an NPM project + Basic Scripting
"This post will walk you through the basic of getting around NPM project, from initializing a project and using custom scripts."
To initialize an NPM project, cd
into the root of your project directory and run:
npm init
package name
is your project's name (default to folder name).entry point
is the file that should be run to start the application (e.g.app.js
,index.js
, etc.)- By default, no script will be generated. You need to write the
start
yourself.
NPM Scripts
To write additional npm scripts, go into the newly generated package.json
file and edit the "scripts": {}
object.
{
"name": "my_node_package",
"version": "1.0.0",
"description": "Just a simple node project.",
"main": "./app.js",
"scripts": { "start": "node ./app.js", "test": "echo \\"Error: no test specified\\" && exit 1", "foo": "echo bar" }, "author": "Romson Preechawit (Woods)",
"license": ""
}
To run the script, do:
npm run foo
npm start
start
is the only script that can be run using the shorthand npm start
without adding the run
command. Other scripts need to use the full npm run <SCRIPT_NAME>
syntax.
npm start
Passing arguments to an NPM scripts
To pass arguments to a script, do:
npm run <SCRIPT_NAME> -- <ARGUMENTS>
For example, in the case of "build": "ng build"
, we can do:
npm run build -- --prod
NPM Dependencies Management
npm install <PACKAGE_NAME>
Common options:
--save-dev
only save this in development dependencies--save
will save for production as well-g
will install the package globally. You normally don't want to do this as the dependencies should be encapsulated within the project itself.
For example:
npm install nodemon --save-dev
This will add these line in our package.json
"devDependencies": {
"nodemon": "^2.0.4"
}